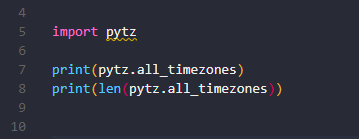
To get a python timezone list, we can use the powerful pytz library. If you do not have pytz installed you can do so by using the pip install pytz command at the command line. Of course you must have Python and pip installed beforehand.
We will be using Python 3.8.10 in this tutorial.
import pytz
print(pytz.all_timezones)
#Outputs
#['Africa/Abidjan', 'Africa/Accra', 'Africa/Addis_Ababa', 'Africa/Algiers', 'Africa/Asmara'...'UTC', #'Universal', 'W-SU', 'WET', 'Zulu']
Using pytz.all_timezones will give you a very long list of type LazyList of World Time Zones with their long names. There is another way we can accomplish this. See below:
import pytz
print(pytz.all_timezones_set)
#Outputs
#LazySet({'Africa/Windhoek', 'Asia/Tashkent', 'Egypt', 'America/Santiago'...'Australia/Yancowinna', #'America/Mazatlan'})
Using pytz.all_timezones_set will give you a long set of type LazySet of World Time Zones with their long names.
There is a third way that we can get a list of timezones. See below:
import pytz
print(pytz.common_timezones)
#Outputs
#['Africa/Abidjan', 'Africa/Accra', 'Africa/Addis_Ababa', 'Africa/Algiers'...'US/Mountain', 'US/Pacific', 'UTC']
Using pytz.common_timezones will give us a slightly smaller LazyList of the more common world timezones.
The first two timezone lists have the same amount of elements (593) but the common_timezones list has 439 elements.
import pytz
print(len(pytz.all_timezones_set))
print(len(pytz.all_timezones))
print(len(pytz.common_timezones))
#Outputs
#593
#593
#439
We can take this a step further and use the pandas package to output any of these lists to a csv file for further reference. We can easily install pandas with the command pip install pandas at the command line and execute the following code. We will attempt to save the pytz.all_timezones_set set to a CSV file on disk:
import pandas as pd Â
import pytz
      Â
df = pd.DataFrame(pytz.all_timezones_set)Â
   Â
#Saving DataFrame to Local Disk and Folder as CSV file
df.to_csv('AllTZ.csv')Â
The above code should save a CSV file with 2 columns and 594 rows (the first row can be deleted) to the disk in your working directory with the name AllTZ.csv. Below is a pic of what that list would look like in MS Excel:
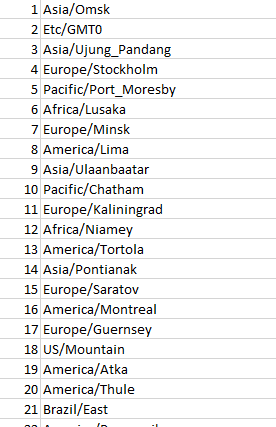
We hope this article helped you to be able to acquire a list of time zones in python.
You can find the documentation for the pytz library HERE.
You can find the documentation for the pandas library HERE.
We also have a searchable list of time zones in python (common) with Long Name and UTC Offset HERE. Thanks for reading! 👌👌👌