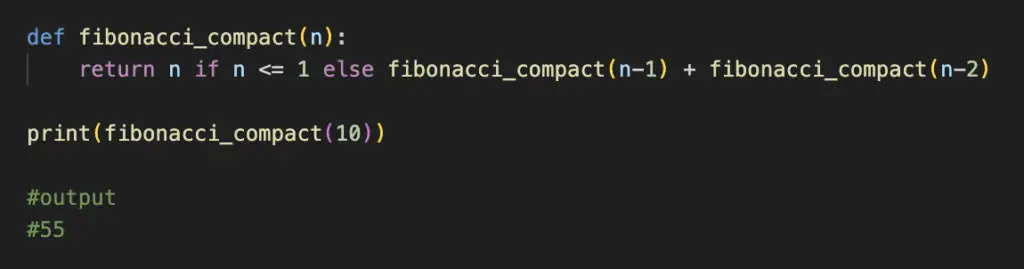
Letβs do a Python Fibonacci Recursive Solution. Letβs go! π₯π₯π₯
The Fibonacci sequence is a series of numbers in which each number (Fibonacci number) is the sum of the two preceding ones. The sequence commonly starts with 0 and 1, although it can also start with 1 and 1. The simplest form of the sequence is:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, β¦
The Fibonacci sequence has various applications and appears in many different areas of mathematics and science, including computer science, mathematics, and even biology.
In this tutorial we will do two examples of the Python Fibonacci recursive solution. A more readable solution and one with minimum lines.
More Readable Solution
This version of the Fibonacci sequence solution focuses on readability. It clearly demonstrates the recursive nature of the problem, where each number in the sequence is the sum of the two preceding ones:
def fibonacci_verbose(n):
# Base case: return 0 or 1 for the first two numbers in the sequence
if n == 0:
return 0
elif n == 1:
return 1
# Recursive case: calculate the sum of the two preceding numbers
else:
return fibonacci_verbose(n-1) + fibonacci_verbose(n-2)
print(fibonacci_verbose(10))
#output
#55
In this implementation, the function fibonacci_verbose takes an integer n and returns the nth number in the Fibonacci sequence. The base case handles the first two numbers of the sequence, 0 and 1, corresponding to n == 0 and n == 1, respectively. The recursive case calls the function itself twice, for n-1 and n-2, and adds their results. This structure makes it very clear how the Fibonacci sequence builds up from its base cases.
Minimum Lines Solution
This version of the Fibonacci sequence solution is condensed into as few lines as possible, while still maintaining the core recursive logic:
def fibonacci_compact(n):
return n if n <= 1 else fibonacci_compact(n-1) + fibonacci_compact(n-2)
print(fibonacci_compact(10))
#output
#55
The fibonacci_compact function is a streamlined version where the entire logic is condensed into a single line. It uses a ternary conditional operator to return n directly if n is less than or equal to 1, which covers the base cases of 0 and 1. If n is greater than 1, it performs the recursive calls to fibonacci_compact(n-1) and fibonacci_compact(n-2) and returns their sum. This compact version provides the same functionality but in a more concise form, suitable for situations where brevity is preferred over explicit clarity.
Thanks for reading out Python Fibonacci Recursive Solution tutorial. Happy coding!ππ»ππ»ππ»