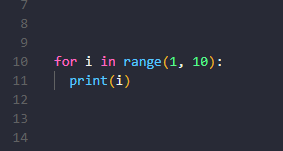
This ‘for i in range Python‘ tutorial will use Python 3.8.10.
To properly explain the ‘for i in range Python’ topic we need to explain 2 concepts:
- The ‘for loop’
- The ‘range’ sequence In Python
For Loops
A loop is a control structure in a software program that is used to repeat a set of instructions multiple times.
Each pass over the complete set of instructions to be repeated is called an ITERATION.
A ‘for loop’ is a particular type of loop. The ‘for loop’ is used to repeat a section of statements for a known or finite number of times. The exact number of times may not always be known to the programmer directly but the CPU executing the code will know. A ‘for loop’ will not be suitable for all situations that require loops.
‘For loops’ are best for iterating over sequences (which can be python lists, tuples and strings) of finite length. In Python, a sequence is an ordered set of items.
The ‘for loop’ will execute the specified set of statements once for each item or member of the sequence starting at the first element in the sequence and ending at the last element of the sequence.
Below is a simple ‘for loop’ in Python 3.
noble_gases = ["helium","neon","argon","krypton","xenon","radon","ununoctium"]
for n in noble_gases:
  print(n)
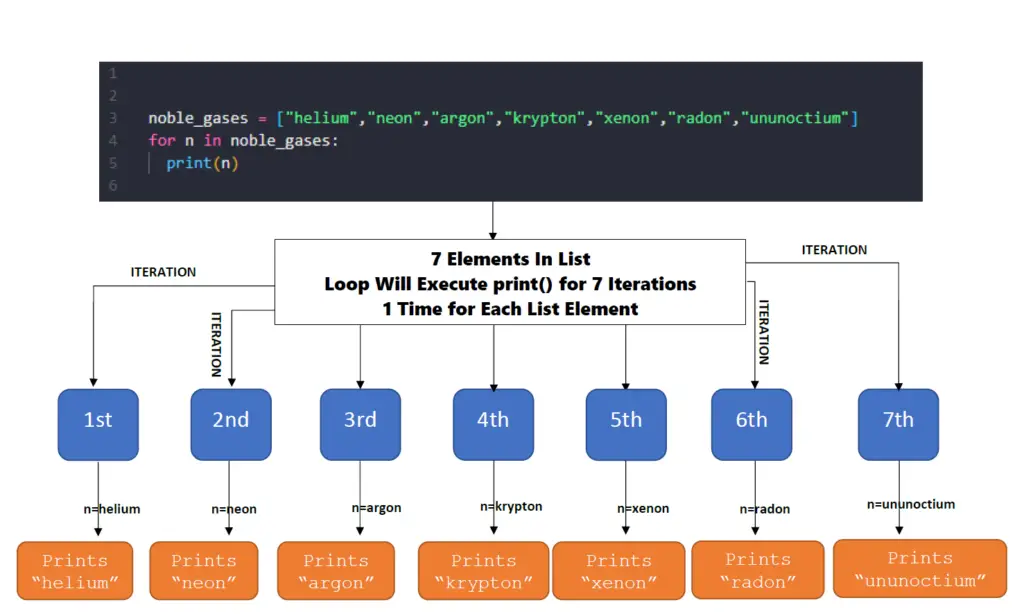
The above diagram explains how our simple ‘for loop’ will execute. Seven elements means that the loop will execute the print statement 7 times.
The sequence noble_gases is a python list.
The n is a variable used to store an individual element of the list. It starts at the first element in the list (helium) and on the next iteration the n will store the next value in the list (neon). This pattern will repeat until the loop reaches the end of the list.
Our output will look like this:
#Output:
#helium
#neon
#argon
#krypton
#xenon
#radon
#ununoctium
Find out more about ‘for loops’ HERE.
range()
Range is actually a class that represents a sequence of numbers. Find documentation HERE.
range() is the range class constructor. We can use range in a few ways but at the very least we must give a stop value for the range constructor in the form range(stop). Where stop is usually an integer. If there isn’t a start parameter, the sequence will start from 0 by default.
For our coming examples we will use the list() constructor which accepts our range() constructor and returns a list which we can print.
print(list(range(10)))
#outputs: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
In one line we can initialize a range, cast it as a list and print it to the console.
We can also give a start and stop value in the form range(start, stop).
print(list(range(1, 10)))
#outputs: [1, 2, 3, 4, 5, 6, 7, 8, 9]
Note the behavior of the range() constructor. It gives us a sequence of values that are inclusive of the start value but excludes the stop value. We define 10 as the stop value but it isn’t actually printed in the result. This is important, please remember this.
Yet another way we can use the range() constructor is range(start, stop, step). The step parameter can be a positive or negative integer.
Where the step is positive, each item in the range is calculated with the formula r[i] = start + step * i where i >= 0 and r[i] < stop.
r[i] refers to an element in the sequence defined by the range() constructor. The r refers to the range sequence and the i is the index of the item in the sequence. The index starts at 0 and increments by 1 for each item in the range object. Therefore r[0] would be the 1st element of the sequence (in the below example that will be the start parameter, 3), r[1] is the second (6), r[2] is the third and so on, as long as r[i] is less than the stop parameter. In the below example the final item of the sequence will be r[9]. Recall that the stop value is NOT included in the output of range.
print(list(range(3, 33, 3)))
#outputs: [3, 6, 9, 12, 15, 18, 21, 24, 27, 30]
The above sequence starts at 3 and increments by 3 until the sequence gets to 33, but it does not actually print this stop item (which in this case is 33).
Because the increment is positive, the above formula will apply. For our first element r[0], the value of i will be 0 so the formula will be: 3+3*0. Which resolved to 3+0 or 3.
For the second element in the sequence, i is equal to 1 so this will resolve to 6 and so on for each additional element of the range.
print(list(range(3, -33, -3))
#output: [3, 0, -3, -6, -9, -12, -15, -18, -21, -24, -27, -30]
The step in the above example is negative so the formula in this case will be r[i] = start + step*i, where i >= 0 and r[i] > stop. For our first element r[0], the value of i will be 0 so the formula will be: 3+(-3)*0. Which resolved to 3-0 or 3.
The second element in the sequence will be 0 because, 3-3*1 resolves to 0. The third element will be -3 because 3-3*2 resolves to -3. This is how it continues until the end of the sequence. Next we move on to the meat of this ‘for i in range Python’ topic.
for i in range()
When we combine the ‘for loop’ and range class object we are actually executing the statements defined in the loop for each member of the range sequence. Recall our example and diagram for how for loops execute, the same will apply here. For example:
for i in range(10):
  print(i)
#Outputs
#0
#1
#2
#3
#4
#5
#6
#7
#8
#9
So our code above prints each element of the range sequence on a new line. i is similar to n in our first diagram and example. i is the variable that will store the element of the sequence currently being processed. Its value will change to the next element of the sequence on the next iteration.
Recall also that it will not print the stop value of 10, as usual. Because we did not give a start parameter, the sequence started from 0. Let’s do another example:
for i in range(1, 10):
  print(i)
#outputs
#1
#2
#3
#4
#5
#6
#7
#8
#9
Note that in this example we have a start value so the sequence starts from that value, which is 1 in this case. Let’s do two more:
for i in range(3, 33, 3):
  print(i)
#Output
#3
#6
#9
#12
#15
#18
#21
#24
#27
#30
Just as we saw previously, when the step (3 in this case) is positive, we will increment by that value according to the formula.
Similarly, when the increment is negative we will increment by that value according to the previously defined formula, as we will see below:
for i in range(3, -33, -3):
  print(i)
#Output:
#3
#0
#-3
#-6
#-9
#-12
#-15
#-18
#-21
#-24
#-27
#-30
Conclusion
The ‘for i in range(x)‘ construct is versatile indeed. We can do any number of steps or statements in our for loops to accomplish many different things according to the needs of our project. Take a look at our final example:
from datetime import *
for i in range(3, 33, 3):
    dt= datetime.now() + timedelta(days=i)
    futureDate = datetime.strptime(str(dt), '%Y-%m-%d %H:%M:%S.%f')
    futureDateFmt=futureDate.strftime("%a %d %b %Y %I:%M:%S.%f %p")
    print(f"{i} days in the future will be {futureDateFmt}")
#Output
#3 days in the future will be Fri 11 Jun 2021 03:06:13.831557 PM
#6 days in the future will be Mon 14 Jun 2021 03:06:13.844499 PM
#9 days in the future will be Thu 17 Jun 2021 03:06:13.845492 PM
#12 days in the future will be Sun 20 Jun 2021 03:06:13.846504 PM
#15 days in the future will be Wed 23 Jun 2021 03:06:13.846504 PM
#18 days in the future will be Sat 26 Jun 2021 03:06:13.847489 PM
#21 days in the future will be Tue 29 Jun 2021 03:06:13.847489 PM
#24 days in the future will be Fri 02 Jul 2021 03:06:13.848485 PM
#27 days in the future will be Mon 05 Jul 2021 03:06:13.848485 PM
#30 days in the future will be Thu 08 Jul 2021 03:06:13.849484 PM
We used the for i in range(x) construct creatively here. We used it to give us a print out of the day and time for every 3rd day for the next 30 days. We also used the datetime library, which you can find a tutorial for HERE. We hope the above tutorial for ‘for i in range Python’ helped. Cheers! 👌👌👌