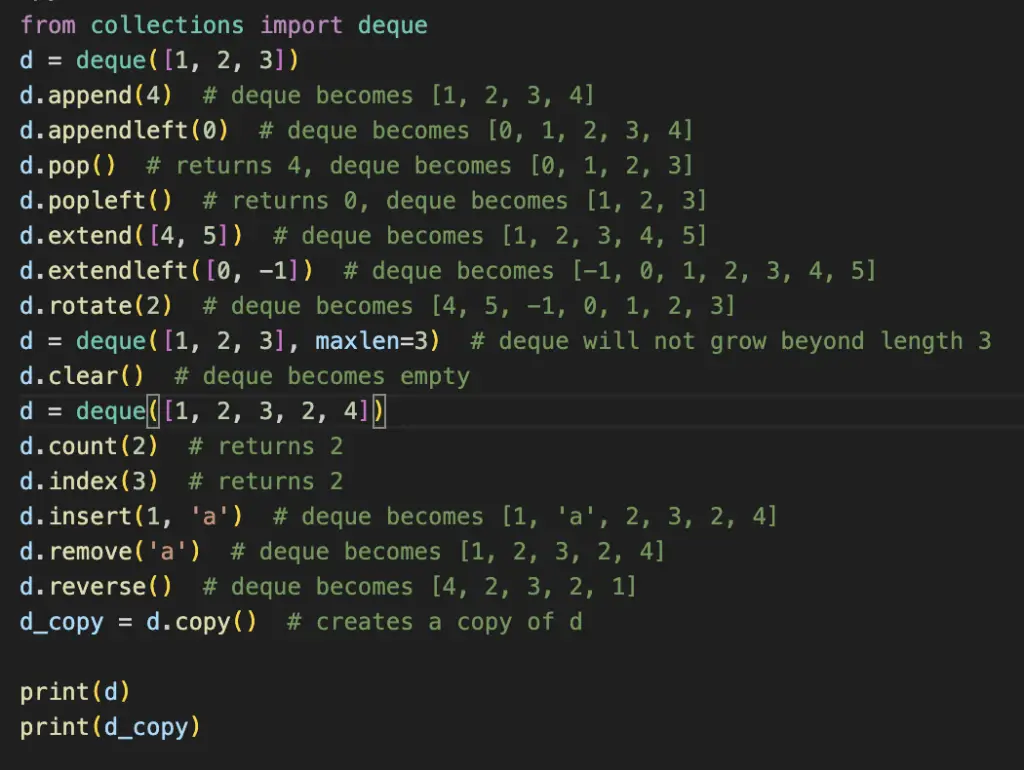
In this post we’ll list Python Deque Methods. Ready? Let’s go! 🔥🔥🔥
A deque (double-ended queue) in Python is a type of list where elements can be added to or removed from both ends (front and back) efficiently. It is part of the collections module and is a more generalized form of stack and queue. Deques are thread-safe and can be used to implement both stacks and queues without the performance penalties associated with lists.
Here’s a list of the key methods used on a deque in Python, along with example code and a short description for each of the Python Deque Methods:
1. append(x) – Adds x to the right side of the deque.
from collections import deque
d = deque([1, 2, 3])
d.append(4) # deque becomes [1, 2, 3, 4]
2. appendleft(x) – Adds x to the left side of the deque.
d.appendleft(0) # deque becomes [0, 1, 2, 3, 4]
3. pop() – Removes and returns an element from the right side of the deque. Raises IndexError if empty.
d.pop() # returns 4, deque becomes [0, 1, 2, 3]
4. popleft() – Removes and returns an element from the left side of the deque. Raises IndexError if empty.
d.popleft() # returns 0, deque becomes [1, 2, 3]
5. extend(iterable) – Adds elements from iterable to the right side of the deque.
d.extend([4, 5]) # deque becomes [1, 2, 3, 4, 5]
6. extendleft(iterable) – Adds elements from iterable to the left side of the deque. Note that the iterable is added in reverse order.
d.extendleft([0, -1]) # deque becomes [-1, 0, 1, 2, 3, 4, 5]
7. rotate(n) – Rotate the deque n steps to the right. If n is negative, rotate to the left.
d.rotate(2) # deque becomes [4, 5, -1, 0, 1, 2, 3]
8. maxlen – Maximum size of a deque or None if unbounded.
d = deque([1, 2, 3], maxlen=3) # deque will not grow beyond length 3
9. clear() – Removes all elements from the deque.
d.clear() # deque becomes empty
10. count(x) – Count the number of deque elements equal to x.
d = deque([1, 2, 3, 2, 4])
d.count(2) # returns 2
11. index(x, [start, [stop]]) – Return the position of x in the deque (at or after index start and before index stop). Raises ValueError if not found.
d.index(3) # returns 2
12. insert(i, x) – Insert x into the deque at position i.
d.insert(1, 'a') # deque becomes [1, 'a', 2, 3, 2, 4]
13. remove(value) – Remove the first occurrence of value. Raises ValueError if not found.
d.remove('a') # deque becomes [1, 2, 3, 2, 4]
14. reverse() – Reverse the elements of the deque in-place.
d.reverse() # deque becomes [4, 2, 3, 2, 1]
15.copy() – Creates a shallow copy of the deque.
d_copy = d.copy() # creates a copy of d
So there you have it, all 15 Python Deque Methods. The full code is as follows:
from collections import deque
d = deque([1, 2, 3])
d.append(4) # deque becomes [1, 2, 3, 4]
d.appendleft(0) # deque becomes [0, 1, 2, 3, 4]
d.pop() # returns 4, deque becomes [0, 1, 2, 3]
d.popleft() # returns 0, deque becomes [1, 2, 3]
d.extend([4, 5]) # deque becomes [1, 2, 3, 4, 5]
d.extendleft([0, -1]) # deque becomes [-1, 0, 1, 2, 3, 4, 5]
d.rotate(2) # deque becomes [4, 5, -1, 0, 1, 2, 3]
d = deque([1, 2, 3], maxlen=3) # deque will not grow beyond length 3
d.clear() # deque becomes empty
d = deque([1, 2, 3, 2, 4])
d.count(2) # returns 2
d.index(3) # returns 2
d.insert(1, 'a') # deque becomes [1, 'a', 2, 3, 2, 4]
d.remove('a') # deque becomes [1, 2, 3, 2, 4]
d.reverse() # deque becomes [4, 2, 3, 2, 1]
d_copy = d.copy() # creates a copy of d
print(d)
print(d_copy)