Let’s explain Python multiline comment syntax and identify some uses. Let’s go! 🔥🔥🔥
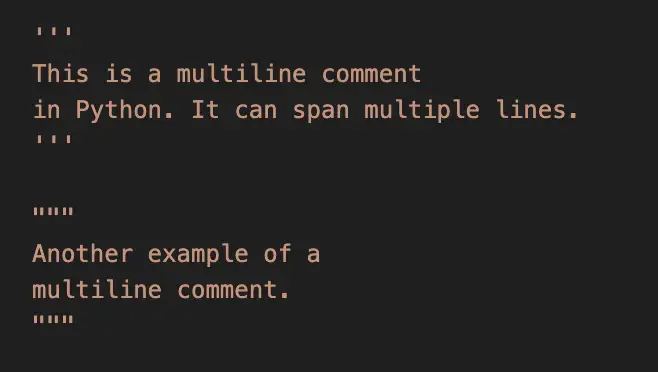
In Python, a multiline comment is created by enclosing the comment text within triple quotes. These can be either triple single quotes ('''
) or triple double quotes ("""
). Anything inside these triple quotes is considered a comment and is not executed by Python. Here’s an example:
'''
This is a multiline comment
in Python. It can span multiple lines.
'''
"""
Another example of a
multiline comment.
"""
These are often used for documentation strings (docstrings) as well, but they can be used anywhere in the code to add explanatory text or temporarily disable blocks of code.
Multiline comments as docstrings can be used to describe the functionality of Python classes, functions, modules, and methods. They’re written in triple quotes and are located just below the definition of a function, class, or module. Here are three examples, ranging from simple to more complex:
def add_numbers(a, b):
"""
Add two numbers and return the result.
Parameters:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of a and b.
"""
return a + b
This example shows a docstring for a class, including its constructor and method:
class Calculator:
"""
A simple calculator class to perform basic arithmetic operations.
Attributes:
history (list): Stores a history of calculations.
"""
def __init__(self):
"""
Initializes the calculator with an empty history.
"""
self.history = []
def add(self, a, b):
"""
Adds two numbers and stores the result in history.
Parameters:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of a and b.
"""
result = a + b
self.history.append(("add", a, b, result))
return result
This is a more complex example of a module docstring, documenting the purpose of the module and its contents:
"""
This module provides advanced mathematical operations and utilities.
It includes classes and functions for handling complex arithmetic operations,
algebraic equations, and numerical analysis. It is designed to be a comprehensive
toolkit for scientific computing.
Classes:
- ComplexNumber: Represents and manipulates complex numbers.
- AlgebraSolver: Solves various algebraic equations.
Functions:
- integrate(func, lower, upper): Numerically integrates a function over a given range.
- differentiate(func, at_point): Calculates the derivative of a function at a given point.
"""
# ... implementation of classes and functions ...
In each of these examples, the docstring provides a clear description of what the function, class, or module does, its parameters, return values, and any other relevant information. This is crucial for anyone who will use or maintain the code in the future.
Once you define your multiline comment docstring, you can then access it using .__doc__ function:
def add_numbers(a, b):
"""
Add two numbers and return the result.
Parameters:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of a and b.
"""
return a + b
#using the .__doc__ built-in function
print(add_numbers.__doc__)
#Will print the following:
# Add two numbers and return the result.
#
# Parameters:
# a (int): The first number.
# b (int): The second number.
#
# Returns:
# int: The sum of a and b.
If you are using Python at the terminal you can use the help(example_function) to access the docstring.
Thanks for reading our Python multiline comment syntax tutorial! Be sure to check out our other great tutorials HERE. 👌🏻👌🏻👌🏻