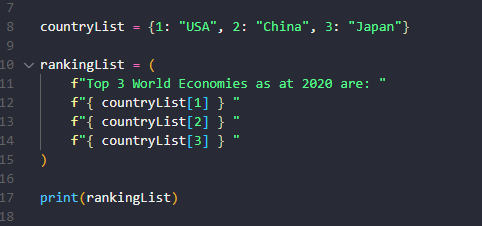
There are many functions in Python 3 that allow us to easily obtain a properly formatted string in python. By strings we actually mean string literals or string variables which comprise numbers, letters, special characters or a combination of all three.
First, let us itemize 3 reasons why formatting is critical:
- Formatting is needed for presentations and reports to peers, middle and upper management in organizations.
- Formatting is needed for mass publications.
- Formatting is needed for better end user experience in applications.
- Formatting is needed within the code itself to make data easier to work with.
Essentially, formatting adjusts string literals and variables (data) to make them more MEANINGFUL, READABLE and/or UNDERSTANDABLE, to the consumers of said data.
To be specific, the consumers can be humans or functions within the code itself, as we will soon see.
Information is a source of learning. But unless it is organized, processed, and available to the right people in a format for decision making, it is a burden, not a benefit.
William Pollard
I strongly recommend you set up a working Python 3 development environment on your workstation to test the below examples of getting a good string format in Python. We will be using Python 3.8.10 for this tutorial.
Let us begin!
SECTIONS (click to go to section)
F-Strings
Numbers/Decimals
Bonus
F-Strings
Starting with Python 3.6 we can use F-strings to output a formatted string in Python. We denote F-strings simply by using the f”” syntax. We put the text we wish to format in between the double quotes.
The F in f-strings means “formatted strings”. The f-string is probably the function that we will use most often when you are trying to get formatted strings in Python.
The biggest benefit of this function is that it allows us to combine expressions in our string literals in a quick and convenient way with little extra coding. Check out the PEP (Python Enhancement Proposal) HERE.
Here are some quick facts about F-strings:
- Evaluated at runtime.
- Very fast.
- Does not work in Python 2.
NOTE: I actually tried this on Python 2.7.13 and, as expected, I got an SyntaxError: invalid syntax Error.
Below are some code examples:
#Snippet 1: use variables and do arithmetic
name = "King"
width = 76
length = 80
print(f"The dimensions of a {name} Sized bed is {width}x{length} inches")
print(f"The dimensions of a {name} Sized bed is {width*2.54}x{length*2.54} centimeters")
print("\n\n") #new line
#Output
#The dimensions of a King Sized bed is 76x80 inches
#The dimensions of a King Sized bed is 193.04x203.2 centimeters
##################################
#Snippet 2: call functions
def farenheit_to_celsius(input):
return ((input - 32) * 5/9)
farenheit = 90
print(f"{farenheit}F converted to celsius is {round(farenheit_to_celsius(farenheit),2)}°")
farenheit = 70
print(f"{farenheit}F converted to celsius is {round(farenheit_to_celsius(farenheit),2)}°")
print("\n\n") #new line
#Output
#90F converted to celsius is 32.22°
#70F converted to celsius is 21.11°
##################################
In the first two example we show how f-strings can be used for variables, arithmetic and to call functions very easily to evaluate within the f-string. Be sure to note the output in each case to get an understanding of how f-strings work to format our string literals dynamically.
#Snippet 3:simple dates
import datetime
cometName = 'comet 13p/olbers'
desc='Halley-type'
passingDate = datetime.date(2024, 6, 30)
#!r allows us to print quotes
print(f'A {desc!r} comet called {cometName.upper()}, will next pass earth on {passingDate:%A, %B %d, %Y}.')
#Output
#A 'Halley-type' comet called COMET 13P/OLBERS, will next pass earth on Sunday, June 30, 2024.
#################################
#Snippet 4: quotation marks (don't use the same inner and enclosing brackets)
print(f'California is nicknamed the {"Golden State"!r}')
print(f"California is nicknamed the {'Golden State'!r}")
#Output
#California is nicknamed the 'Golden State'
#California is nicknamed the 'Golden State'
#################################
#Snippet 5: Curly Brackets
print(f"4 ∉ {{2,3,5,7,11}}")
x='[3 + (2x – 1) + x] – 2'
print(f"Simplify: 5 + 2{{{x}}}")
print(f"{{{1,2,3,4,5}}}")
print("\n")
#Output
#4 ∉ {2,3,5,7,11}
#Simplify: 5 + 2{[3 + (2x – 1) + x] – 2}
#{(1, 2, 3, 4, 5)}
#################################
In the above snippets we see examples of case conversion, the use of dates, quotation marks and curly brackets. All very simple use cases of the f-string, all of which allow us to get a formatted string in python.
###################################
#Snippet 6
countryList = {
1: "USA",
2: "China",
3: "Japan"
}
rankingList = (
f"Top 3 World Economies as at 2020 are: "
f"{countryList[1]} "
f"{countryList[2]} "
f"{countryList[3]} "
)
print(rankingList)
print("\n")
#Output
#Top 3 World Economies as at 2020 are: USA China Japan
#####################################
#Snippet 7: Multiple lines
#the above didn't give us what we expected so we can do this
#using "" gives us exact formatting, line by line
betterRankingList = (
f"""
Top 3 World Economies by Size in 2020 are:
{countryList[1]}
{countryList[2]}
{countryList[3]}
"""
)
print(betterRankingList)
print("\n")
#Output
#Top 3 World Economies by Size in 2020 are:
#USA
#China
#Japan
#####################################################################################
Pay special attention to the above! Do you see how we can use f-strings to format LAYOUT aside from the actual text itself? This is a POWERFUL tool that can supercharge your skills! ⚡⚡⚡ Let’s do some more! 🔥🔥🔥
#Snippet 8: More Layout Formatting
countryGDPList = {
"USA": "22,675,271",
"China":"16,642,318",
"Japan":"5,378,136"
}
#The :<X Left aligns output within the available space specified by X characters eg. :<20 left align within 20 character space.
betterRankingList = (
f"""
Top 3 World Economies by GDP (2021 est.) are:
{'COUNTRY':<10} {'GDP (US$ M) per IMF':<20}
{'USA':<11}{countryGDPList["USA"]:<20}
{'China':<11}{countryGDPList["China"]:<20}
{'Japan':<11}{countryGDPList["Japan"]:<20}
"""
)
print(betterRankingList)
print("\n")
#Output
#Top 3 World Economies by GDP (2021 est.) are:
# COUNTRY GDP (US$ M) per IMF
# USA 22,675,271
# China 16,642,318
# Japan 5,378,136
##############################################
#Snippet 9: More Layout Formatting
#The :>X Right aligns output within the available space specified by X characters eg. :>20 right align within 20 character space.
betterRankingList = (
f"""
Top 3 World Economies by GDP (2021 est.) are:
{'COUNTRY':<10} {'GDP (US$ M) per IMF':>20}
{'USA':<11}{countryGDPList["USA"]:>20}
{'China':<11}{countryGDPList["China"]:>20}
{'Japan':<11}{countryGDPList["Japan"]:>20}
"""
)
print(betterRankingList)
print("\n")
#Output
#Top 3 World Economies by GDP (2021 est.) are:
# COUNTRY GDP (US$ M) per IMF
# USA 22,675,271
# China 16,642,318
# Japan 5,378,136
##############################################
#Snippet 10: More Layout Formatting
#The :^X Center aligns output within the available space specified by X characters eg. :^20 center align within 20 character space.
betterRankingList = (
f"""
Top 3 World Economies by GDP (2021 est.) are:
{'COUNTRY':<10} {'GDP (US$ M) per IMF':^20}
{'USA':<11}{countryGDPList["USA"]:^20}
{'China':<11}{countryGDPList["China"]:^20}
{'Japan':<11}{countryGDPList["Japan"]:^20}
"""
)
print(betterRankingList)
print("\n")
#Top 3 World Economies by GDP (2021 est.) are:
# COUNTRY GDP (US$ M) per IMF
# USA 22,675,271
# China 16,642,318
# Japan 5,378,136
##############################################
Ah! Now we have more tools that we can use. Formatting types like :< , :> and :^ are powerful tools we can use to format the layout of our strings. Find the complete list HERE.
Numbers/Decimals
What if we are working on a project and we are presented with a dataset or user input field where there are string literals that are decimals and they look something like this:
‘3248935030.778899’
What is that? A dollar amount or something else? We must format this string properly first. We must first get the output of getcontext().
#Snippet 11: Decimals
import locale
from decimal import *
print(getcontext())
#Output:
#Context(prec=28, rounding=ROUND_HALF_EVEN, Emin=-999999, Emax=999999, capitals=1, clamp=0, flags=[],
#traps=[InvalidOperation, DivisionByZero, Overflow])
########################################
You can find more information HERE. For the purposes of this tutorial we are only interested in prec and rounding for now. By setting prec and rounding we can get some consistency in all of our calculations.
#Snippet 12: More Decimals
import locale
from decimal import *
#round takes 2 arguments:
#number and digits
#number = number to be rounded
#digits = decimal places
print(round(3248935030.778899,2))
#or simple conversion from string to decimal
newFloat = float("3248935030.778899")
#check type
print(type(newFloat))
#Output
#3248935030.78
#<class 'float'>
######################################
#Snippet 13
#set precision
getcontext().prec = 4
#whoa!
print(12.3456*65.4321)
#Output
#807.79853376
#####################################
#Snippet 14
#won't work, even worse actually!
print(Decimal(12.345*65.432))
#Output
#807.7580400000000508953235112130641937255859375
#####################################
#Snippet 15
#works! much better!
print(f"{Decimal(12.345)*Decimal(65.432)}")
print(f"{Decimal('12.345')*Decimal('65.432')}")
#Output
#807.8
#807.8
#####################################
#Snippet 16
#set rounding
getcontext().rounding = 'ROUND_CEILING'
print(Decimal(22) / Decimal(7))
print(Decimal(1) / Decimal(3))
#Output
#3.143
#0.3334
#####################################
#Snippet 17
#set rounding
getcontext().rounding = 'ROUND_FLOOR'
print(Decimal(22) / Decimal(7))
print(Decimal(1) / Decimal(3))
#Output
#3.142
#0.3333
#####################################
#Snippet 18: Locale
#locale based decimal systems
#USA: using :n formatting type
locale.setlocale(locale.LC_NUMERIC, "en_US")
print(f'{Decimal("5000000.00"):n}')
#Germany
locale.setlocale(locale.LC_NUMERIC, "de_DE")
print(f'{Decimal("5000000.00"):n}')
print(f'{Decimal("0.1234"):n}')
#Switzerland
locale.setlocale(locale.LC_NUMERIC, "gsw_CH")
print(f'{Decimal("5000000.00"):n}')
print(f'{Decimal("0.1234"):n}')
#India
locale.setlocale(locale.LC_NUMERIC, "ta_IN")
print(f'{Decimal("5000000.00"):n}')
print(f'{Decimal("0.1234"):n}')
#Output
#5,000,000.00
#5.000.000,00
#0,1234
#5’000’000.00
#0.1234
#50,00,000.00
#0.1234
#####################################
Pay special attention to Snippet 15. The Decimal() function does implicit conversion if the string literal is already a number. If it isn’t a number or contains letters or other non-numbers you will get an InvalidOperation error.
Look at Snippet 18. See how locale is important? Keep this in mind developers! Always keep in mind who your audience is and where they will be. This is another important part of getting a properly formatted string in python.
NOTE: In this section we used locale codes. The “en_US” and “de_DE” are examples of this. There is a pretty complete list of locale codes that you can find HERE.
Bonus
Yes, it is possible to add color with Python 3 to the console! 🙌🙌 First, we install these three libraries which can be found HERE, HERE and HERE:
pip install colorama
pip install termcolor
pip install prompt-toolkit
#Snippet 26
from colorama import *
from termcolor import colored
from prompt_toolkit import print_formatted_text, HTML
init(autoreset=True)
print(Fore.RED + 'some red text')
print(Style.BRIGHT + Fore.RED + 'some bright red text')
print(colored('Hello, World!', 'green', 'on_red'))
print_formatted_text(HTML('<b>This is bold</b>'))
print_formatted_text(HTML('<i>This is italic</i>'))
print_formatted_text(HTML('<u>This is underlined</u>'))
#Output
#some red text
#some bright red text
#Hello, World!
#This is bold
#This is italic
#This is underlined
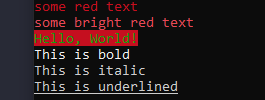
Beautiful isn’t it? Not only can we format the type of data and the layout but also the color and graphic look too! Awesome! 😜😜 See how powerful Python is?
CLICK HERE FOR PART 2 OF OUR TUTORIAL. We hope you found the article useful for your project. You might want to bookmark this article and use a reference for many years to come on your Python 3 journey! A properly formatted string in python will make all the difference to your audience, users, reviews etc. Thanks for sticking with us. 👌👌